Typescript Classes
ES2015 Classes are syntatic sugar to the JavaScript prototypal inheritance model. This JavaScript pattern of simmulating class-like inheritance, provides the benefits of object-oriented techniques fundamental to the creation of reusable components. ES2015 Classes in terms of OOP (object-oriented programing) is a blue print to create new objects.
TypeScript type annotations provides more expressive relationships between classes.
To declare a class, we can use the class
keyword.
// By convention we capitalize the name of the class. class User {} // Instace of the class const user = new User()
Constructor
We use a constructor method to inizialize an object with a class.
class User { first_name: string last_name: string constructor(first_name: string, last_name: string) { this.first_name = first_name this.last_name = last_name } }
By prefixing a parameter property with a one of the access modifiers (public, private or protected) TypeScript allow us to simplify the three declarations into one line.
class User { constructor(public first_name: string, public last_name: string) {} }
Access modifiers
Access modifiers change the visibility of the properties and methods of a class.
TypeScript provides three access modifiers: public, private, and protected.
Public: The public modifier allows properties and methods to be accessible from anywhere. This is also the default modifier.
class User { // Public modifier constructor(public first_name: string, public last_name: string) {} preferredName: string = this.first_name // Getter method. get fullname() { return `${this.first_name} ${this.last_name}` } // Setter method. set addPreferredName(name: string) { this.preferredName = name } } const student = new User("John", "Doe") console.log(student.fullname) // John Doe console.log(student.preferName) // John student.addPreferName = "Jonny" console.log(student.preferName) // Jonny
Private: The private modifier cannot be accessed from outside of its containing class. Similarly, static methods are called on the class itself, not instances of the class.
class User { constructor(private first_name: string, private last_name: string) {} get fullname() { return `${this.first_name} ${this.last_name}` } } const student = new User("John", "Doe") console.log(student.fullname) // John Doe console.log(student.first_name) /* Property 'first_name' is private and only accessible within class 'User'. */
Protected: A Protected access modifier can be accessed only within the class and its subclasses.
class User { constructor( public first_name: string, public last_name: string, protected age: number ) {} } class Student extends User { get isAdult(): boolean { return this.age >= 21 } } const student = new Student("John", "Doe", 16) console.log(student.isAdult) // false console.log(student.age) /* Property 'age' is protected and only accessible within class 'User' and its subclasses. */
Inheritance
To create a class inheritance, use the extends
keyword. In the above example, Student extends the functionality of User.
We can overwrite methods by redefining the method inside the subclass.
class User { constructor( public first_name: string, public last_name: string, protected age: number ) {} get fullname() { return `${this.first_name} ${this.last_name}` } get isAdult(): boolean { return this.age >= 21 } } class Student extends User { get isAdult(): boolean { return this.age >= 18 } } const student = new Student("John", "Doe", 18) console.log(student.isAdult) //true
When adding a constructor in the subclasses. We must use super()
. The super
keyword refers to the parent class. It is used to call the constructor of the parent class and to access the parent's properties and methods.
class Student extends User { // Don't need the modifier when params belong to the parent class constructor( first_name: string, last_name: string, age: number, public honors: boolean ) { // Reference to contructor method in the parent class super(first_name, last_name, age) } get isAdult(): boolean { return this.age >= 18 } get getAvailableActivities() { return this.honors ? "a, b, c" : null } } const studentA = new Student("John", "Doe", 18, true) console.log(studentA.fullname) // John Doe console.log(studentA.isAdult) // true console.log(studentA.getAvailableActivities) // a, b, c const studentB = new Student("Jane", "Smith", 16, false) console.log(studentB.fullname) // Jane Smith console.log(studentB.isAdult) // false console.log(studentB.getAvailableActivities) // null const faculty = new User("Mario", "Ponce", 32) console.log(faculty.fullname) // Mario Ponce console.log(faculty.isAdult) // True
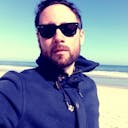
Hey, I'm Ignacio Villamar
Senior Frontend Engineer, living in the NYC metro area.
Follow @ivstudio