As frontend developers, we often need to transform and optimize design assets to fit properly into our libraries or frameworks. For example, when using SVGs in React, we typically convert them into functional components, which allows for reusability and easier customization, like adjusting sizes or changing fill colors.
In this article, I will share how I automate the conversion of SVGs into React components effortlessly using SVGR to build an Avatar NPM package for React.
Tyger Avatar NPMWhat is SVGR?
SVGR is a universal tool that transforms SVGs into React components. It takes a raw SVG and converts it into a ready-to-use React component. For my project, I used the CLI configuration to transform an entire directory.
Configuration
- Install SVGR CLI:
npm i -D @svgr/cli
- Next, we need to add the SVGR script to our
package.json
.
"svgr": "svgr --icon --title-prop --no-dimensions --typescript -d src/TrAvatars assets",
svgr
: Runs thesvgr
tool.icon
: Treats SVGs as icons, setting width and height to1em
.title-prop
: Adds atitle
prop for accessibility.no-dimensions
: Removes width and height to allow CSS styling.typescript
: Generates TypeScript components.d src/TrAvatars
: Sets output directory tosrc/TrAvatars
.assets
: Input directory for SVG files.
There are many customization options to optimize our generated React component.
SVGR Template
The SVGR template allow us to optionally customize the final generated React component.
View template customization options
const propTypesTemplate = ( { imports, interfaces, componentName, props, jsx, exports }, { tpl } ) => { return tpl`${imports} ${interfaces} function ${componentName}(${props}) { return ${jsx}; } ${exports} `; }; module.exports = propTypesTemplate;
Create an .svgrrc.js
file in the root directory to inform SVGR about the file template.
module.exports = { template: require('./svgr-template'), };
SVGR Transformation
We can convert our svgs to react components by running: npm run svgr
├── assets │ ├── SampleOne.svg │ └── SampleTwo.svg ├── src | |-- TrAvatars | | |-- SvgSampleOne.tsx | | |-- SvgSampleTwo.tsx | | └── index.tsx └── package.json
- Input: Link to my assets
- Output: Link to my generated React Components
Tyger Avatar
Using SVGR to automate the transformation of SVGs into React components significantly streamlined my workflow. By applying batch transformations through a consistent SVGR template, I maintained uniformity across all components without manual updates. This method is especially beneficial for teams managing large asset collections.
For Tyger Avatar, I used Rollup and the @svgr/rollup
plugin for bundling the project. However, there is support for most popular bundlers, libraries, and frameworks. Check out the SVGR documentation for more details.
Additionally, I implemented unit tests with React Testing Library to maintain high-quality standards and documented the functionality with a Storybook page. View Source Code.
StorybookIllustrations
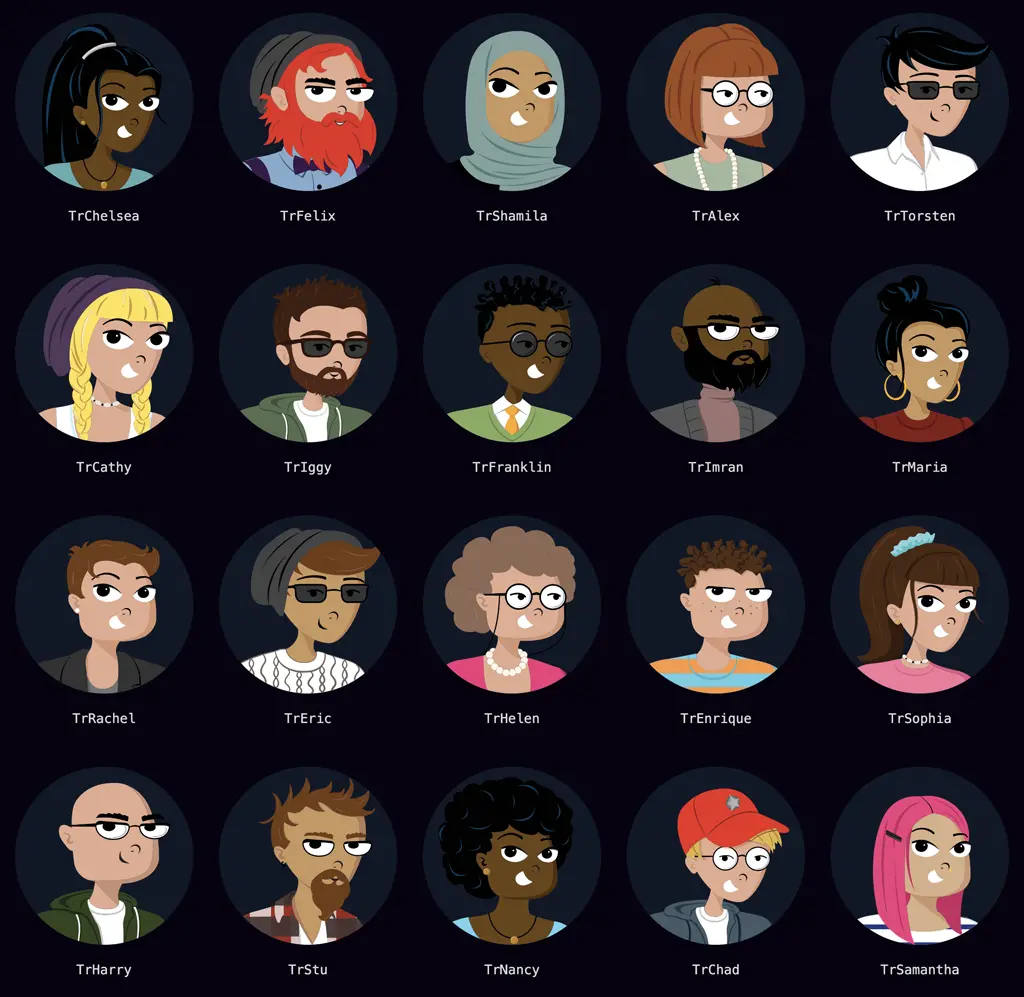
Avatar illustrations by Cathy Villamar, view the the character design process.